Java编程中最基础的文件和目录操作方法详解
文件操作
平常经常使用JAVA对文件进行读写等操作,这里汇总一下常用的文件操作。
1、创建文件
public static boolean createFile(String filePath){ boolean result = false; File file = new File(filePath); if(!file.exists()){ try { result = file.createNewFile(); } catch (IOException e) { e.printStackTrace(); } } return result; }
2、创建文件夹
public static boolean createDirectory(String directory){ boolean result = false; File file = new File(directory); if(!file.exists()){ result = file.mkdirs(); } return result; }
3、删除文件
public static boolean deleteFile(String filePath){ boolean result = false; File file = new File(filePath); if(file.exists() && file.isFile()){ result = file.delete(); } return result; }
4、删除文件夹
递归删除文件夹下面的子文件和文件夹
public static void deleteDirectory(String filePath){ File file = new File(filePath); if(!file.exists()){ return; } if(file.isFile()){ file.delete(); }else if(file.isDirectory()){ File[] files = file.listFiles(); for (File myfile : files) { deleteDirectory(filePath + "/" + myfile.getName()); } file.delete(); } }
5、读文件
(1)以字节为单位读取文件,常用于读二进制文件,如图片、声音、影像等文件
public static String readFileByBytes(String filePath){ File file = new File(filePath); if(!file.exists() || !file.isFile()){ return null; } StringBuffer content = new StringBuffer(); try { byte[] temp = new byte[1024]; FileInputStream fileInputStream = new FileInputStream(file); while(fileInputStream.read(temp) != -1){ content.append(new String(temp)); temp = new byte[1024]; } fileInputStream.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } return content.toString(); }
(2)以字符为单位读取文件,常用于读文本,数字等类型的文件,支持读取中文
public static String readFileByChars(String filePath){ File file = new File(filePath); if(!file.exists() || !file.isFile()){ return null; } StringBuffer content = new StringBuffer(); try { char[] temp = new char[1024]; FileInputStream fileInputStream = new FileInputStream(file); InputStreamReader inputStreamReader = new InputStreamReader(fileInputStream, "GBK"); while(inputStreamReader.read(temp) != -1){ content.append(new String(temp)); temp = new char[1024]; } fileInputStream.close(); inputStreamReader.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } return content.toString(); }
(3)以行为单位读取文件,常用于读面向行的格式化文件
public static List<String> readFileByLines(String filePath){ File file = new File(filePath); if(!file.exists() || !file.isFile()){ return null; } List<String> content = new ArrayList<String>(); try { FileInputStream fileInputStream = new FileInputStream(file); InputStreamReader inputStreamReader = new InputStreamReader(fileInputStream, "GBK"); BufferedReader reader = new BufferedReader(inputStreamReader); String lineContent = ""; while ((lineContent = reader.readLine()) != null) { content.add(lineContent); System.out.println(lineContent); } fileInputStream.close(); inputStreamReader.close(); reader.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } return content; }
6、写文件
字符串写入文件的几个类中,FileWriter效率最高,BufferedOutputStream次之,FileOutputStream最差。
(1)通过FileOutputStream写入文件
public static void writeFileByFileOutputStream(String filePath, String content) throws IOException{ File file = new File(filePath); synchronized (file) { FileOutputStream fos = new FileOutputStream(filePath); fos.write(content.getBytes("GBK")); fos.close(); } }
(2)通过BufferedOutputStream写入文件
public static void writeFileByBufferedOutputStream(String filePath, String content) throws IOException{ File file = new File(filePath); synchronized (file) { BufferedOutputStream fos = new BufferedOutputStream(new FileOutputStream(filePath)); fos.write(content.getBytes("GBK")); fos.flush(); fos.close(); } }
(3)通过FileWriter将字符串写入文件
public static void writeFileByFileWriter(String filePath, String content) throws IOException{ File file = new File(filePath); synchronized (file) { FileWriter fw = new FileWriter(filePath); fw.write(content); fw.close(); } }
目录操作
目录是一个文件可以包含其他文件和目录的列表。你想要在目录中列出可用文件列表,可以通过使用 File 对象创建目录,获得完整详细的能在 File 对象中调用的以及有关目录的方法列表。
创建目录
这里有两个有用的文件方法,能够创建目录:
mkdir( ) 方法创建了一个目录,成功返回 true ,创建失败返回 false。失败情况是指文件对象的路径已经存在了,或者无法创建目录,因为整个路径不存在。
mkdirs( ) 方法创建一个目录和它的上级目录。
以下示例创建 “/ tmp / user / java / bin” 目录:
import java.io.File; public class CreateDir { public static void main(String args[]) { String dirname = "/tmp/user/java/bin"; File d = new File(dirname); // Create directory now. d.mkdirs(); } }
编译并执行以上代码创建 “/ tmp /user/ java / bin”。
提示:Java 自动按 UNIX 和 Windows 约定来处理路径分隔符。如果在 Windows 版本的 Java 中使用正斜杠(/),仍然可以得到正确的路径。
目录列表
如下,你能够用 File 对象提供的 list() 方法来列出目录中所有可用的文件和目录
import java.io.File; public class ReadDir { public static void main(String[] args) { File file = null; String[] paths; try{ // create new file object file = new File("/tmp"); // array of files and directory paths = file.list(); // for each name in the path array for(String path:paths) { // prints filename and directory name System.out.println(path); } }catch(Exception e){ // if any error occurs e.printStackTrace(); } } }
基于你/ tmp目录下可用的目录和文件,将产生以下结果:
test1.txt test2.txt ReadDir.java ReadDir.class
栏 目:JAVA代码
本文地址:http://www.codeinn.net/misctech/13051.html
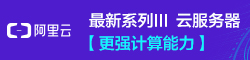
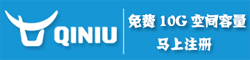
阅读排行
- 1Java Swing组件BoxLayout布局用法示例
- 2java中-jar 与nohup的对比
- 3Java邮件发送程序(可以同时发给多个地址、可以带附件)
- 4Caused by: java.lang.ClassNotFoundException: org.objectweb.asm.Type异常
- 5Java中自定义异常详解及实例代码
- 6深入理解Java中的克隆
- 7java读取excel文件的两种方法
- 8解析SpringSecurity+JWT认证流程实现
- 9spring boot里增加表单验证hibernate-validator并在freemarker模板里显示错误信息(推荐)
- 10深入解析java虚拟机