图书管理系统java代码实现
本文实例为大家分享了java实现图书管理系统的具体代码,供大家参考,具体内容如下
/* (程序头部注释开始)
* 程序的版权和版本声明部分
* Copyright (c) 2011, 烟台大学计算机学院学生
* All rights reserved.
* 文件名称: 《图书管理系统――java》
* 作 者: 刘江波
* 完成日期: 2012 年 3 月 1 日
* 版 本 号: v3.0
* 对任务及求解方法的描述部分
* 问题描述:
* 程序头部的注释结束
*/
文件包的建立情况:
BookDao.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ package com.liu.dao; import com.liu.po.BookBean; import java.io.*; import java.util.HashMap; import java.util.Map; import java.util.logging.Level; import java.util.logging.Logger; /** * * @author asus */ public class BookDAO { // 写 public void writeBook(Map<Integer,BookBean >bookMap){ // FileOutputStream fos = null; ObjectOutputStream oos = null; try { fos = new FileOutputStream("F:\\缓存区\\book.txt"); oos = new ObjectOutputStream(fos); oos.writeObject(bookMap); //清空缓存区 oos.flush(); } catch (FileNotFoundException ex) { Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); } //异常级别高的在后边 catch (IOException ex) { Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); } finally{ try{ //先开后闭 oos.close(); fos.close(); }catch(IOException ex){ Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE,null,ex); } } } //读 public Map<Integer,BookBean>readBook(){ FileInputStream fis = null; ObjectInputStream ois = null; Map<Integer, BookBean> map = null; try { fis = new FileInputStream("F:\\缓存区\\book.txt"); ois = new ObjectInputStream(fis); map = (Map<Integer, BookBean>) ois.readObject();//出现异常进入catch } catch (ClassNotFoundException ex) { Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); } catch (FileNotFoundException ex) { Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); } catch (IOException ex) { //Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); //ex.printStackTrace(); map=new HashMap<Integer,BookBean>();//出现异常时,进行创建map } finally{ try { if(ois!=null){ ois.close(); } if(fis!=null){ fis.close(); } } catch (IOException ex) { Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); } } return map; } }
TypeDao.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ package com.liu.dao; import com.liu.po.TypeBean; import java.io.*; import java.util.*; import java.util.logging.*; /** * * 对文件进行读和写操作 */ public class TypeDAO { // 写 public void writeType(Map<Integer,TypeBean >typeMap){ // FileOutputStream fos = null; ObjectOutputStream oos = null; try { fos = new FileOutputStream("F:\\缓存区\\type.txt"); oos = new ObjectOutputStream(fos); oos.writeObject(typeMap); //清空缓存区 oos.flush(); } catch (FileNotFoundException ex) { Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); } //异常级别高的在后边 catch (IOException ex) { Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); } finally{ try{ //先开后闭 oos.close(); fos.close(); }catch(IOException ex){ Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE,null,ex); } } } //读 public Map<Integer,TypeBean>readType(){ FileInputStream fis = null; ObjectInputStream ois = null; Map<Integer, TypeBean> map = null; try { fis = new FileInputStream("F:\\缓存区\\type.txt"); ois = new ObjectInputStream(fis); map = (Map<Integer, TypeBean>) ois.readObject();//出现异常进入catch } catch (ClassNotFoundException ex) { Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); } catch (FileNotFoundException ex) { Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); } catch (IOException ex) { //Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); //ex.printStackTrace(); map=new HashMap<Integer,TypeBean>();//出现异常时,进行创建map } finally{ try { if(ois!=null){ ois.close(); } if(fis!=null){ fis.close(); } } catch (IOException ex) { Logger.getLogger(TypeDAO.class.getName()).log(Level.SEVERE, null, ex); } } return map; } }
BookBean.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ package com.liu.po; import java.io.Serializable; /** * * @author asus */ public class BookBean implements Serializable{ private int id; private String bookName; private String bookType; private String memo; private String money; /** * @return the id */ public int getId() { return id; } /** * @param id the id to set */ public void setId(int id) { this.id = id; } /** * @return the bookName */ public String getBookName() { return bookName; } /** * @param bookName the bookName to set */ public void setBookName(String bookName) { this.bookName = bookName; } /** * @return the bookType */ public String getBookType() { return bookType; } /** * @param bookType the bookType to set */ public void setBookType(String bookType) { this.bookType = bookType; } /** * @return the memo */ public String getMemo() { return memo; } /** * @param memo the memo to set */ public void setMemo(String memo) { this.memo = memo; } /** * @return the money */ public String getMoney() { return money; } /** * @param money the money to set */ public void setMoney(String money) { this.money = money; } }
TypeBean.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ package com.liu.po; /** * * @author asus */ import java.io.Serializable; public class TypeBean implements Serializable{ private int id; private String typeName; private String memo; /** * @return the id */ public int getId() { return id; } /** * @param id the id to set */ public void setId(int id) { this.id = id; } /** * @return the typeName */ public String getTypeName() { return typeName; } /** * @param typeName the typeName to set */ public void setTypeName(String typeName) { this.typeName = typeName; } /** * @return the memo */ public String getMemo() { return memo; } /** * @param memo the memo to set */ public void setMemo(String memo) { this.memo = memo; } }
LoginForm.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ /* * LoginForm.java * * Created on 2013-2-26, 18:33:36 */ package com.liu.view; import java.awt.event.KeyAdapter; import java.awt.event.KeyEvent; import javax.swing.JOptionPane; /** * * @author asus */ public class LoginForm extends javax.swing.JFrame { /** Creates new form LoginForm */ public LoginForm() { initComponents(); } /** This method is called from within the constructor to * initialize the form. * WARNING: Do NOT modify this code. The content of this method is * always regenerated by the Form Editor. */ @SuppressWarnings("unchecked") // <editor-fold defaultstate="collapsed" desc="Generated Code"> private void initComponents() { jLabel1 = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); jLabel3 = new javax.swing.JLabel(); LoginName = new javax.swing.JTextField(); LoginPwd = new javax.swing.JPasswordField(); jButton1 = new javax.swing.JButton(); setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE); setTitle("登陆界面"); setBounds(new java.awt.Rectangle(300, 200, 0, 0)); setIconImage(new javax.swing.ImageIcon(getClass().getResource("/com/liu/resouce/logo.jpg")).getImage()); addKeyListener(new java.awt.event.KeyAdapter() { public void keyPressed(java.awt.event.KeyEvent evt) { formKeyPressed(evt); } }); jLabel1.setFont(new java.awt.Font("宋体", 0, 36)); jLabel1.setForeground(new java.awt.Color(204, 0, 0)); jLabel1.setText("图书管理系统"); jLabel2.setFont(new java.awt.Font("宋体", 0, 24)); jLabel2.setText("用户名:"); jLabel3.setFont(new java.awt.Font("宋体", 0, 24)); jLabel3.setText("密码:"); LoginName.setName(""); // NOI18N LoginName.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { LoginNameActionPerformed(evt); } }); LoginName.addKeyListener(new java.awt.event.KeyAdapter() { public void keyPressed(java.awt.event.KeyEvent evt) { LoginNameKeyPressed(evt); } }); LoginPwd.addKeyListener(new java.awt.event.KeyAdapter() { public void keyPressed(java.awt.event.KeyEvent evt) { LoginPwdKeyPressed(evt); } }); jButton1.setFont(new java.awt.Font("宋体", 0, 24)); // NOI18N jButton1.setText("登录"); jButton1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton1ActionPerformed(evt); } }); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(97, 97, 97) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jLabel2) .addComponent(jLabel3)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false) .addComponent(LoginPwd) .addComponent(LoginName, javax.swing.GroupLayout.DEFAULT_SIZE, 215, Short.MAX_VALUE)) .addContainerGap(88, Short.MAX_VALUE)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addContainerGap(130, Short.MAX_VALUE) .addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 263, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(113, 113, 113)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addContainerGap(299, Short.MAX_VALUE) .addComponent(jButton1, javax.swing.GroupLayout.PREFERRED_SIZE, 97, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(110, 110, 110)) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(50, 50, 50) .addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 45, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(38, 38, 38) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel2) .addComponent(LoginName, javax.swing.GroupLayout.PREFERRED_SIZE, 29, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(26, 26, 26) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel3) .addComponent(LoginPwd, javax.swing.GroupLayout.PREFERRED_SIZE, 29, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(18, 18, 18) .addComponent(jButton1, javax.swing.GroupLayout.PREFERRED_SIZE, 36, javax.swing.GroupLayout.PREFERRED_SIZE) .addContainerGap(21, Short.MAX_VALUE)) ); pack(); }// </editor-fold> private void LoginNameActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: } private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) { //1.先获取用户名和密码 String name = LoginName.getText(); String password = new String(LoginPwd.getPassword()); //2.进行验证 if("admin".equals(name)&&"admin".equals(password)) { //登陆成功 //隐藏LoginForm,显示MainForm this.setVisible(false); new MainForm().setVisible(true); } else { //登录失败 JOptionPane.showMessageDialog(this, "用户名或密码错误!"); } } private void formKeyPressed(java.awt.event.KeyEvent evt) { //敲击键盘登陆 } private void LoginNameKeyPressed(java.awt.event.KeyEvent evt) { //敲击键盘登陆 if(evt.getKeyText(evt.getKeyCode()).compareToIgnoreCase("Enter")==0) { jButton1.doClick(); } } private void LoginPwdKeyPressed(java.awt.event.KeyEvent evt) { //敲击键盘登陆 if(evt.getKeyText(evt.getKeyCode()).compareToIgnoreCase("Enter")==0) { jButton1.doClick(); } } /** * @param args the command line arguments */ public static void main(String args[]) { java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new LoginForm().setVisible(true); } }); } // Variables declaration - do not modify private javax.swing.JTextField LoginName; private javax.swing.JPasswordField LoginPwd; private javax.swing.JButton jButton1; private javax.swing.JLabel jLabel1; private javax.swing.JLabel jLabel2; private javax.swing.JLabel jLabel3; // End of variables declaration }
MainForm.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ /* * MainForm.java * * Created on 2013-2-26, 18:35:25 */ package com.liu.view; /** * * @author asus */ public class MainForm extends javax.swing.JFrame { /** Creates new form MainForm */ public MainForm() { initComponents(); } /** This method is called from within the constructor to * initialize the form. * WARNING: Do NOT modify this code. The content of this method is * always regenerated by the Form Editor. */ @SuppressWarnings("unchecked") // <editor-fold defaultstate="collapsed" desc="Generated Code"> private void initComponents() { jLabel1 = new javax.swing.JLabel(); jPanel1 = new javax.swing.JPanel(); jLabel2 = new javax.swing.JLabel(); jMenuBar1 = new javax.swing.JMenuBar(); 配置管理 = new javax.swing.JMenu(); jMenuItem1 = new javax.swing.JMenuItem(); jMenuItem2 = new javax.swing.JMenuItem(); jMenu2 = new javax.swing.JMenu(); jMenuItem4 = new javax.swing.JMenuItem(); jMenu1 = new javax.swing.JMenu(); jMenuItem3 = new javax.swing.JMenuItem(); setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE); setTitle("图书管理系统"); setBounds(new java.awt.Rectangle(80, 60, 0, 0)); setIconImage(new javax.swing.ImageIcon(getClass().getResource("/com/liu/resouce/logo.jpg")).getImage()); jLabel1.setFont(new java.awt.Font("宋体", 0, 48)); jLabel1.setForeground(new java.awt.Color(0, 204, 51)); jLabel1.setIcon(new javax.swing.ImageIcon(getClass().getResource("/com/liu/resouce/main.jpg"))); // NOI18N jLabel2.setFont(new java.awt.Font("宋体", 0, 48)); jLabel2.setForeground(new java.awt.Color(0, 0, 255)); jLabel2.setText("欢迎使用图书借阅管理系统"); javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1); jPanel1.setLayout(jPanel1Layout); jPanel1Layout.setHorizontalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGap(38, 38, 38) .addComponent(jLabel2) .addContainerGap(63, Short.MAX_VALUE)) ); jPanel1Layout.setVerticalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel1Layout.createSequentialGroup() .addContainerGap() .addComponent(jLabel2, javax.swing.GroupLayout.DEFAULT_SIZE, 78, Short.MAX_VALUE)) ); 配置管理.setText("配置管理"); jMenuItem1.setAccelerator(javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_L, java.awt.event.InputEvent.CTRL_MASK)); jMenuItem1.setText("类别管理"); jMenuItem1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem1ActionPerformed(evt); } }); 配置管理.add(jMenuItem1); jMenuItem2.setAccelerator(javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_T, java.awt.event.InputEvent.CTRL_MASK)); jMenuItem2.setText("图书管理"); jMenuItem2.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem2ActionPerformed(evt); } }); 配置管理.add(jMenuItem2); jMenuBar1.add(配置管理); jMenu2.setText("借书"); jMenuItem4.setAccelerator(javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_J, java.awt.event.InputEvent.CTRL_MASK)); jMenuItem4.setText("租书"); jMenu2.add(jMenuItem4); jMenuBar1.add(jMenu2); jMenu1.setText("还书"); jMenuItem3.setAccelerator(javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_H, java.awt.event.InputEvent.CTRL_MASK)); jMenuItem3.setText("还书"); jMenu1.add(jMenuItem3); jMenuBar1.add(jMenu1); setJMenuBar(jMenuBar1); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jLabel1, 0, 0, Short.MAX_VALUE) .addGroup(layout.createSequentialGroup() .addGap(22, 22, 22) .addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addContainerGap()) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addContainerGap() .addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 411, javax.swing.GroupLayout.PREFERRED_SIZE)) ); pack(); }// </editor-fold> private void jMenuItem1ActionPerformed(java.awt.event.ActionEvent evt) { // 类型管理 new TypeForm().setVisible(true); } private void jMenuItem2ActionPerformed(java.awt.event.ActionEvent evt) { // 图书管理 new BookForm().setVisible(true); } /** * @param args the command line arguments */ public static void main(String args[]) { java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new MainForm().setVisible(true); } }); } // Variables declaration - do not modify private javax.swing.JLabel jLabel1; private javax.swing.JLabel jLabel2; private javax.swing.JMenu jMenu1; private javax.swing.JMenu jMenu2; private javax.swing.JMenuBar jMenuBar1; private javax.swing.JMenuItem jMenuItem1; private javax.swing.JMenuItem jMenuItem2; private javax.swing.JMenuItem jMenuItem3; private javax.swing.JMenuItem jMenuItem4; private javax.swing.JPanel jPanel1; private javax.swing.JMenu 配置管理; // End of variables declaration }
BookForm.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ /* * BookForm.java * * Created on 2013-2-28, 8:23:01 */ package com.liu.view; import com.liu.dao.BookDAO; import com.liu.dao.TypeDAO; import com.liu.po.BookBean; import com.liu.po.TypeBean; import java.util.Map; import java.util.Set; import java.util.Vector; import javax.swing.DefaultComboBoxModel; import javax.swing.JOptionPane; import javax.swing.table.DefaultTableModel; /** * * @author asus */ public class BookForm extends javax.swing.JFrame { /** Creates new form BookForm */ private Map<Integer,BookBean> map; private Map<Integer,TypeBean> map1; private BookDAO bookDao; private TypeDAO typeDao; public BookForm() { initComponents(); bookDao = new BookDAO(); typeDao = new TypeDAO(); map = bookDao.readBook(); map1 = typeDao.readType(); initType(); initData(); } /** This method is called from within the constructor to * initialize the form. * WARNING: Do NOT modify this code. The content of this method is * always regenerated by the Form Editor. */ @SuppressWarnings("unchecked") // <editor-fold defaultstate="collapsed" desc="Generated Code"> private void initComponents() { jPanel1 = new javax.swing.JPanel(); jScrollPane1 = new javax.swing.JScrollPane(); bookTable = new javax.swing.JTable(); jPanel2 = new javax.swing.JPanel(); bmemo = new javax.swing.JTextField(); jButton1 = new javax.swing.JButton(); jButton2 = new javax.swing.JButton(); jButton3 = new javax.swing.JButton(); jButton4 = new javax.swing.JButton(); jLabel1 = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); jLabel3 = new javax.swing.JLabel(); jLabel4 = new javax.swing.JLabel(); bid = new javax.swing.JTextField(); bname = new javax.swing.JTextField(); btype = new javax.swing.JComboBox(); jLabel5 = new javax.swing.JLabel(); bmoney = new javax.swing.JTextField(); setTitle("图书管理"); setBounds(new java.awt.Rectangle(100, 50, 0, 0)); setIconImage(new javax.swing.ImageIcon(getClass().getResource("/com/liu/resouce/logo.jpg")).getImage()); bookTable.setFont(new java.awt.Font("宋体", 0, 18)); // NOI18N bookTable.setModel(new javax.swing.table.DefaultTableModel( new Object [][] { {null, null, null, null, null}, {null, null, null, null, null}, {null, null, null, null, null}, {null, null, null, null, null}, {null, null, null, null, null}, {null, null, null, null, null}, {null, null, null, null, null}, {null, null, null, null, null}, {null, null, null, null, null}, {null, null, null, null, null} }, new String [] { "序号", "图书名称", "图书类型", "租金", "备注" } ) { Class[] types = new Class [] { java.lang.Integer.class, java.lang.String.class, java.lang.String.class, java.lang.String.class, java.lang.String.class }; boolean[] canEdit = new boolean [] { false, false, false, false, false }; public Class getColumnClass(int columnIndex) { return types [columnIndex]; } public boolean isCellEditable(int rowIndex, int columnIndex) { return canEdit [columnIndex]; } }); bookTable.addMouseListener(new java.awt.event.MouseAdapter() { public void mouseClicked(java.awt.event.MouseEvent evt) { bookTableMouseClicked(evt); } }); jScrollPane1.setViewportView(bookTable); jPanel2.setBorder(javax.swing.BorderFactory.createTitledBorder(null, "详细信息", javax.swing.border.TitledBorder.DEFAULT_JUSTIFICATION, javax.swing.border.TitledBorder.DEFAULT_POSITION, new java.awt.Font("宋体", 0, 18))); // NOI18N bmemo.setFont(new java.awt.Font("宋体", 0, 18)); jButton1.setText("新增"); jButton1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton1ActionPerformed(evt); } }); jButton2.setText("保存"); jButton2.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton2ActionPerformed(evt); } }); jButton3.setText("更新"); jButton3.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton3ActionPerformed(evt); } }); jButton4.setText("删除"); jButton4.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton4ActionPerformed(evt); } }); jLabel1.setFont(new java.awt.Font("宋体", 0, 18)); jLabel1.setText("序号:"); jLabel2.setFont(new java.awt.Font("宋体", 0, 18)); jLabel2.setText("名称:"); jLabel3.setFont(new java.awt.Font("宋体", 0, 18)); jLabel3.setText("类型:"); jLabel4.setFont(new java.awt.Font("宋体", 0, 18)); jLabel4.setText("备注:"); bid.setFont(new java.awt.Font("宋体", 0, 18)); bname.setFont(new java.awt.Font("宋体", 0, 18)); btype.setFont(new java.awt.Font("宋体", 0, 18)); btype.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "文学类", "教育类", "科技类", "文艺类" })); btype.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { btypeActionPerformed(evt); } }); jLabel5.setFont(new java.awt.Font("宋体", 0, 18)); jLabel5.setText("租金:"); javax.swing.GroupLayout jPanel2Layout = new javax.swing.GroupLayout(jPanel2); jPanel2.setLayout(jPanel2Layout); jPanel2Layout.setHorizontalGroup( jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel2Layout.createSequentialGroup() .addGap(33, 33, 33) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel2Layout.createSequentialGroup() .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jLabel2, javax.swing.GroupLayout.DEFAULT_SIZE, 83, Short.MAX_VALUE) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING) .addComponent(jLabel3) .addComponent(jLabel5) .addComponent(jLabel4))) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)) .addGroup(jPanel2Layout.createSequentialGroup() .addComponent(jLabel1) .addGap(33, 33, 33))) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(bmemo, javax.swing.GroupLayout.DEFAULT_SIZE, 375, Short.MAX_VALUE) .addComponent(bid, javax.swing.GroupLayout.DEFAULT_SIZE, 375, Short.MAX_VALUE) .addComponent(bname, javax.swing.GroupLayout.DEFAULT_SIZE, 375, Short.MAX_VALUE) .addComponent(bmoney, javax.swing.GroupLayout.DEFAULT_SIZE, 375, Short.MAX_VALUE) .addGroup(jPanel2Layout.createSequentialGroup() .addComponent(jButton1, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(28, 28, 28) .addComponent(jButton2, javax.swing.GroupLayout.PREFERRED_SIZE, 69, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(30, 30, 30) .addComponent(jButton3, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 28, Short.MAX_VALUE) .addComponent(jButton4, javax.swing.GroupLayout.PREFERRED_SIZE, 74, javax.swing.GroupLayout.PREFERRED_SIZE)) .addComponent(btype, 0, 375, Short.MAX_VALUE)) .addGap(65, 65, 65)) ); jPanel2Layout.setVerticalGroup( jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel2Layout.createSequentialGroup() .addContainerGap() .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 25, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(bid, javax.swing.GroupLayout.PREFERRED_SIZE, 37, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(13, 13, 13) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel2) .addComponent(bname, javax.swing.GroupLayout.PREFERRED_SIZE, 37, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(18, 18, 18) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel3) .addComponent(btype, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(26, 26, 26) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel5) .addComponent(bmoney, javax.swing.GroupLayout.PREFERRED_SIZE, 35, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(32, 32, 32) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel2Layout.createSequentialGroup() .addComponent(bmemo, javax.swing.GroupLayout.PREFERRED_SIZE, 58, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(64, 64, 64) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jButton1, javax.swing.GroupLayout.PREFERRED_SIZE, 32, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton2, javax.swing.GroupLayout.PREFERRED_SIZE, 32, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton3, javax.swing.GroupLayout.PREFERRED_SIZE, 31, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton4, javax.swing.GroupLayout.PREFERRED_SIZE, 32, javax.swing.GroupLayout.PREFERRED_SIZE))) .addComponent(jLabel4)) .addGap(22, 22, 22)) ); javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1); jPanel1.setLayout(jPanel1Layout); jPanel1Layout.setHorizontalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jScrollPane1, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, 795, Short.MAX_VALUE) .addGroup(jPanel1Layout.createSequentialGroup() .addGap(50, 50, 50) .addComponent(jPanel2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE) .addContainerGap(173, Short.MAX_VALUE)) ); jPanel1Layout.setVerticalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 192, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(10, 10, 10) .addComponent(jPanel2, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)) ); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) ); pack(); }// </editor-fold> //表格数据的初始化 public void initData(){ //加载数据 DefaultTableModel dtm = (DefaultTableModel)bookTable.getModel(); //清空表 while(dtm.getRowCount()>0){ dtm.removeRow(0); } //加载数据 Set<Integer>set = map.keySet(); for(Integer i:set){ BookBean bean = map.get(i); Vector v = new Vector(); v.add(bean.getId()); v.add(bean.getBookName()); v.add(bean.getBookType()); v.add(bean.getMoney()); v.add(bean.getMemo()); dtm.addRow(v); } } //获取类别管理的所有类别 public void initType(){ Set<Integer> set = map1.keySet(); DefaultComboBoxModel dcm = (DefaultComboBoxModel)btype.getModel(); dcm.removeAllElements(); for(Integer i:set){ TypeBean bean = map1.get(i); dcm.addElement(bean.getTypeName()); } } private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) { // 保存功能 //先保存文本框里的值 String id = bid.getText(); String bookName = bname.getText(); String bookType = (String) btype.getSelectedItem(); String memo = bmemo.getText(); String money = bmoney.getText(); //封装成对象 BookBean bean = new BookBean(); bean.setId(Integer.parseInt(id)); bean.setBookName(bookName); bean.setBookType(bookType); bean.setMemo(memo); bean.setMoney(money); //将bean放到map里面 // Map<Integer,TypeBean>map = new HashMap<Integer,TypeBean>(); map.put(Integer.parseInt(id), bean); //将map放到文件里面 bookDao.writeBook(map); //刷新table initData(); } private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) { // 新增时,将文本框里的信息进行清空,并将序列号置为可编辑。 bid.setEnabled(true); bid.setText(""); bname.setText(""); btype.setSelectedItem(""); bmemo.setText(""); bmoney.setText(""); } private void bookTableMouseClicked(java.awt.event.MouseEvent evt) { //获取选中行号及序列号 int currentRow = bookTable.getSelectedRow(); //BookBean bean = map.get( currentRow); // 将选中的行,显示到信息栏中 bid.setText((Integer) bookTable.getValueAt(currentRow, 0)+""); bname.setText((String) bookTable.getValueAt(currentRow, 1)); btype.setSelectedItem((String) bookTable.getValueAt(currentRow, 2)); bmoney.setText((String) bookTable.getValueAt(currentRow, 3)); bmemo.setText((String) bookTable.getValueAt(currentRow, 4)); //bmemo.setText(bean.getMemo()); bid.setEnabled(false); //序号框不可编辑 } private void jButton4ActionPerformed(java.awt.event.ActionEvent evt) { // 删除操作 //获取选中行号及序列号 int currentRow = bookTable.getSelectedRow(); int id = (Integer)bookTable.getValueAt(currentRow, 0); map.remove(id); bookDao.writeBook(map); JOptionPane.showMessageDialog(this,"类别删除成功"); initData(); } private void btypeActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: } private void jButton3ActionPerformed(java.awt.event.ActionEvent evt) { // 更新操作 //先保存文本框里的值 int currentRow = bookTable.getSelectedRow(); int id = (Integer) bookTable.getValueAt(currentRow, 0); String bookName = bname.getText(); String bookType = (String) btype.getSelectedItem(); String memo = bmemo.getText(); String money = bmoney.getText(); //封装成对象 BookBean bean = new BookBean(); bean.setId(id); bean.setBookName(bookName); bean.setBookType(bookType); bean.setMemo(memo); bean.setMoney(money); //将bean放到map里面 // Map<Integer,TypeBean>map = new HashMap<Integer,TypeBean>(); map.put(id, bean); //将map放到文件里面 bookDao.writeBook(map); //刷新table JOptionPane.showMessageDialog(this,"类别更新成功"); initData(); } /** * @param args the command line arguments */ public static void main(String args[]) { java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new BookForm().setVisible(true); } }); } // Variables declaration - do not modify private javax.swing.JTextField bid; private javax.swing.JTextField bmemo; private javax.swing.JTextField bmoney; private javax.swing.JTextField bname; private javax.swing.JTable bookTable; private javax.swing.JComboBox btype; private javax.swing.JButton jButton1; private javax.swing.JButton jButton2; private javax.swing.JButton jButton3; private javax.swing.JButton jButton4; private javax.swing.JLabel jLabel1; private javax.swing.JLabel jLabel2; private javax.swing.JLabel jLabel3; private javax.swing.JLabel jLabel4; private javax.swing.JLabel jLabel5; private javax.swing.JPanel jPanel1; private javax.swing.JPanel jPanel2; private javax.swing.JScrollPane jScrollPane1; // End of variables declaration }
TypeForm.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ /* * TypeForm.java * * Created on 2013-2-26, 19:07:51 */ package com.liu.view; import com.liu.dao.TypeDAO; import com.liu.po.TypeBean; import java.util.HashMap; import java.util.Map; import java.util.Set; import java.util.Vector; import javax.swing.JOptionPane; import javax.swing.table.DefaultTableModel; /** * * @author asus */ public class TypeForm extends javax.swing.JFrame { private TypeDAO typeDao; private Map<Integer,TypeBean> map; /** Creates new form TypeForm */ public TypeForm() { initComponents(); typeDao = new TypeDAO(); map = typeDao.readType(); initData(); } /** This method is called from within the constructor to * initialize the form. * WARNING: Do NOT modify this code. The content of this method is * always regenerated by the Form Editor. */ @SuppressWarnings("unchecked") // <editor-fold defaultstate="collapsed" desc="Generated Code"> private void initComponents() { jPanel1 = new javax.swing.JPanel(); jScrollPane1 = new javax.swing.JScrollPane(); typeTable = new javax.swing.JTable(); jPanel2 = new javax.swing.JPanel(); jLabel1 = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); jLabel3 = new javax.swing.JLabel(); tid = new javax.swing.JTextField(); tname = new javax.swing.JTextField(); jScrollPane2 = new javax.swing.JScrollPane(); tmemo = new javax.swing.JTextArea(); jButton1 = new javax.swing.JButton(); jButton3 = new javax.swing.JButton(); jButton4 = new javax.swing.JButton(); jButton5 = new javax.swing.JButton(); setTitle("类型管理"); setBounds(new java.awt.Rectangle(100, 50, 0, 0)); setIconImage(new javax.swing.ImageIcon(getClass().getResource("/com/liu/resouce/logo.jpg")).getImage()); typeTable.setFont(new java.awt.Font("宋体", 0, 18)); typeTable.setModel(new javax.swing.table.DefaultTableModel( new Object [][] { {null, null, null}, {null, null, null}, {null, null, null}, {null, null, null} }, new String [] { "序号", "类别名称", "备注" } ) { Class[] types = new Class [] { java.lang.Integer.class, java.lang.String.class, java.lang.String.class }; boolean[] canEdit = new boolean [] { false, false, false }; public Class getColumnClass(int columnIndex) { return types [columnIndex]; } public boolean isCellEditable(int rowIndex, int columnIndex) { return canEdit [columnIndex]; } }); typeTable.setColumnSelectionAllowed(true); typeTable.addMouseListener(new java.awt.event.MouseAdapter() { public void mouseClicked(java.awt.event.MouseEvent evt) { typeTableMouseClicked(evt); } }); typeTable.addContainerListener(new java.awt.event.ContainerAdapter() { public void componentAdded(java.awt.event.ContainerEvent evt) { typeTableComponentAdded(evt); } }); jScrollPane1.setViewportView(typeTable); typeTable.getColumnModel().getSelectionModel().setSelectionMode(javax.swing.ListSelectionModel.SINGLE_INTERVAL_SELECTION); typeTable.getColumnModel().getColumn(0).setResizable(false); typeTable.getColumnModel().getColumn(2).setResizable(false); jPanel2.setBorder(javax.swing.BorderFactory.createTitledBorder(null, "类别信息", javax.swing.border.TitledBorder.DEFAULT_JUSTIFICATION, javax.swing.border.TitledBorder.DEFAULT_POSITION, new java.awt.Font("宋体", 0, 18))); // NOI18N jLabel1.setFont(new java.awt.Font("宋体", 0, 18)); jLabel1.setText("序号:"); jLabel2.setFont(new java.awt.Font("宋体", 0, 18)); jLabel2.setText("类别名称:"); jLabel3.setFont(new java.awt.Font("宋体", 0, 18)); jLabel3.setText("备注:"); tid.setFont(new java.awt.Font("宋体", 0, 18)); tid.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { tidActionPerformed(evt); } }); tname.setFont(new java.awt.Font("宋体", 0, 18)); tmemo.setColumns(20); tmemo.setFont(new java.awt.Font("Monospaced", 0, 18)); tmemo.setRows(5); jScrollPane2.setViewportView(tmemo); jButton1.setText("保存"); jButton1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton1ActionPerformed(evt); } }); jButton3.setText("更新"); jButton3.addMouseListener(new java.awt.event.MouseAdapter() { public void mouseClicked(java.awt.event.MouseEvent evt) { jButton3MouseClicked(evt); } }); jButton3.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton3ActionPerformed(evt); } }); jButton4.setText("删除"); jButton4.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton4ActionPerformed(evt); } }); jButton5.setText("新增"); jButton5.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton5ActionPerformed(evt); } }); javax.swing.GroupLayout jPanel2Layout = new javax.swing.GroupLayout(jPanel2); jPanel2.setLayout(jPanel2Layout); jPanel2Layout.setHorizontalGroup( jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel2Layout.createSequentialGroup() .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel2Layout.createSequentialGroup() .addGap(39, 39, 39) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel2Layout.createSequentialGroup() .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, 90, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addComponent(tname, javax.swing.GroupLayout.DEFAULT_SIZE, 341, Short.MAX_VALUE)) .addGroup(jPanel2Layout.createSequentialGroup() .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jLabel3) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jScrollPane2, javax.swing.GroupLayout.PREFERRED_SIZE, 383, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGroup(jPanel2Layout.createSequentialGroup() .addComponent(jLabel1) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(tid, javax.swing.GroupLayout.DEFAULT_SIZE, 383, Short.MAX_VALUE)))) .addGroup(jPanel2Layout.createSequentialGroup() .addGap(70, 70, 70) .addComponent(jButton5, javax.swing.GroupLayout.PREFERRED_SIZE, 69, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(41, 41, 41) .addComponent(jButton1, javax.swing.GroupLayout.PREFERRED_SIZE, 68, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(37, 37, 37) .addComponent(jButton3, javax.swing.GroupLayout.PREFERRED_SIZE, 68, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 40, Short.MAX_VALUE) .addComponent(jButton4, javax.swing.GroupLayout.PREFERRED_SIZE, 67, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(20, 20, 20))) .addGap(83, 83, 83)) ); jPanel2Layout.setVerticalGroup( jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel2Layout.createSequentialGroup() .addGap(31, 31, 31) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 21, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(tid, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(27, 27, 27) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, 21, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(tname, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel2Layout.createSequentialGroup() .addGap(45, 45, 45) .addComponent(jLabel3)) .addGroup(jPanel2Layout.createSequentialGroup() .addGap(24, 24, 24) .addComponent(jScrollPane2, javax.swing.GroupLayout.PREFERRED_SIZE, 92, javax.swing.GroupLayout.PREFERRED_SIZE))) .addGap(35, 35, 35) .addGroup(jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jButton5, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton1, javax.swing.GroupLayout.PREFERRED_SIZE, 33, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton3, javax.swing.GroupLayout.PREFERRED_SIZE, 32, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton4, javax.swing.GroupLayout.PREFERRED_SIZE, 33, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(18, 18, 18)) ); javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1); jPanel1.setLayout(jPanel1Layout); jPanel1Layout.setHorizontalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGap(66, 66, 66) .addComponent(jPanel2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE) .addContainerGap(55, Short.MAX_VALUE)) .addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 696, Short.MAX_VALUE) ); jPanel1Layout.setVerticalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 177, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(18, 18, 18) .addComponent(jPanel2, javax.swing.GroupLayout.DEFAULT_SIZE, 361, Short.MAX_VALUE) .addContainerGap()) ); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE) .addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)) ); pack(); }// </editor-fold> //表格数据的初始化 public void initData(){ //加载数据 DefaultTableModel dtm = (DefaultTableModel)typeTable.getModel(); //清空表 while(dtm.getRowCount()>0){ dtm.removeRow(0); } //加载数据 Set<Integer>set = map.keySet(); for(Integer i:set){ TypeBean bean = map.get(i); Vector v = new Vector(); v.add(bean.getId()); v.add(bean.getTypeName()); v.add(bean.getMemo()); dtm.addRow(v); } } private void tidActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: } private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) { //保存类型操作 //先保存文本框里的值 String id = tid.getText(); String typeName = tname.getText(); String memo = tmemo.getText(); //封装成对象 TypeBean bean = new TypeBean(); bean.setId(Integer.parseInt(id)); bean.setMemo(memo); bean.setTypeName(typeName); //将bean放到map里面 // Map<Integer,TypeBean>map = new HashMap<Integer,TypeBean>(); map.put(Integer.parseInt(id), bean); //将map放到文件里面 typeDao.writeType(map); //刷新table initData(); } private void typeTableComponentAdded(java.awt.event.ContainerEvent evt) { // TODO add your handling code here: } private void jButton4ActionPerformed(java.awt.event.ActionEvent evt) { // 删除操作 //获取选中行号及序列号 int currentRow = typeTable.getSelectedRow(); int id = (Integer)typeTable.getValueAt(currentRow, 0); map.remove(id); typeDao.writeType(map); JOptionPane.showMessageDialog(this,"类别删除成功"); initData(); } private void jButton3MouseClicked(java.awt.event.MouseEvent evt) { } private void jButton3ActionPerformed(java.awt.event.ActionEvent evt) { // 更新操作 //先保存文本框里的值 int currentRow = typeTable.getSelectedRow(); int id = (Integer) typeTable.getValueAt(currentRow, 0); String typeName = tname.getText(); String memo = tmemo.getText(); //封装成对象 TypeBean bean = new TypeBean(); bean.setId(id); bean.setMemo(memo); bean.setTypeName(typeName); //将bean放到map里面 // Map<Integer,TypeBean>map = new HashMap<Integer,TypeBean>(); map.put(id, bean); //将map放到文件里面 typeDao.writeType(map); //刷新table JOptionPane.showMessageDialog(this,"类别更新成功"); initData(); } private void typeTableMouseClicked(java.awt.event.MouseEvent evt) { //获取选中行号及序列号 int currentRow = typeTable.getSelectedRow(); // 将选中的行,显示到信息栏中 tid.setText((Integer) typeTable.getValueAt(currentRow, 0)+""); tname.setText((String) typeTable.getValueAt(currentRow, 1)); tmemo.setText((String) typeTable.getValueAt(currentRow, 2)); tid.setEnabled(false); //序号框不可编辑 } private void jButton5ActionPerformed(java.awt.event.ActionEvent evt) { // 新增时,将文本框里的信息进行清空,并将序列号置为可编辑。 tid.setEnabled(true); tid.setText(""); tname.setText(""); tmemo.setText(""); } /** * @param args the command line arguments */ public static void main(String args[]) { java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new TypeForm().setVisible(true); } }); } // Variables declaration - do not modify private javax.swing.JButton jButton1; private javax.swing.JButton jButton3; private javax.swing.JButton jButton4; private javax.swing.JButton jButton5; private javax.swing.JLabel jLabel1; private javax.swing.JLabel jLabel2; private javax.swing.JLabel jLabel3; private javax.swing.JPanel jPanel1; private javax.swing.JPanel jPanel2; private javax.swing.JScrollPane jScrollPane1; private javax.swing.JScrollPane jScrollPane2; private javax.swing.JTextField tid; private javax.swing.JTextArea tmemo; private javax.swing.JTextField tname; private javax.swing.JTable typeTable; // End of variables declaration }
更多学习资料请关注专题《管理系统开发》。
栏 目:JAVA代码
下一篇:Java中的notyfy()和notifyAll()的本质区别
本文标题:图书管理系统java代码实现
本文地址:http://www.codeinn.net/misctech/13309.html
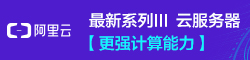
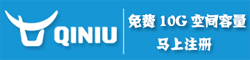
阅读排行
- 1Java Swing组件BoxLayout布局用法示例
- 2java中-jar 与nohup的对比
- 3Java邮件发送程序(可以同时发给多个地址、可以带附件)
- 4Caused by: java.lang.ClassNotFoundException: org.objectweb.asm.Type异常
- 5Java中自定义异常详解及实例代码
- 6深入理解Java中的克隆
- 7java读取excel文件的两种方法
- 8解析SpringSecurity+JWT认证流程实现
- 9spring boot里增加表单验证hibernate-validator并在freemarker模板里显示错误信息(推荐)
- 10深入解析java虚拟机