linq中的分区操作符
Linq中的分区指的是在不重新排列元素的情况下,将输入序列划分为两部分,然后返回其中一个部分的操作。
一、Take操作符
Take(int n)表示将从序列的开头返回数量为n的连续元素,常用于分页。其定义如下:
public static IEnumerable<TSource> Take<TSource>(this IEnumerable<TSource> source, int count);
该方法只接受一个整数,表示要返回的结果的数量。
看下面的例子:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace PartitionOperation { class Program { static void Main(string[] args) { int[] source = new int[] { 86, 2, 77, 94, 100, 65, 5, 22, 70, 55, 81, 66, 45 }; // 返回6个连续的数据 var q = source.Take(6); foreach (var item in q) { Console.WriteLine(item); } Console.ReadKey(); } } }
结果:
二、TakeWhile操作符
TakeWhile操作符用于从输入序列中返回指定数量且满足一定条件的元素。TakeWhile方法执行时将逐个比较序列中的每个元素是否满足指定条件,直到碰到不符合指定条件的元素时,返回前面所有的元素组成的序列。看方法的定义;
public static IEnumerable<TSource> TakeWhile<TSource>(this IEnumerable<TSource> source, Func<TSource, bool> predicate); public static IEnumerable<TSource> TakeWhile<TSource>(this IEnumerable<TSource> source, Func<TSource, int, bool> predicate);
当TakeWhile操作符被调用时,会将source序列中的每一个元素顺序传递给委托predicate,只有那些使得predicate返回值为true的元素才会被添加到结果序列中。要特别注意的是,当TakeWhile操作符在查找过程中,遇到第一个返回false的元素就会立即停止执行,跳出,不管后面还有没有符合条件的元素,即使后面有符合条件的元素也不会要了。对于第二个扩展方法,委托里面含有一个int类型的参数,该参数代表集合的下标,可以依据此进行一些据下标操作的逻辑。
看下面的例子:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace PartitionOperation { class Program { static void Main(string[] args) { int[] source = new int[] { 86, 2, 77, 94, 99, 100, 5, 22, 70, 55, 100, 66, 45 }; // 返回集合中元素值小于100的序列 var q = source.TakeWhile(i => i < 100); foreach (var item in q) { Console.WriteLine(item); } Console.ReadKey(); } } }
结果:
三、Skip操作符
Skip操作符用于从输入序列中跳过指定数量的元素,返回由序列中剩余的元素所组成的新序列。来看下Skip的方法定义:
public static IEnumerable<TSource> Skip<TSource>(this IEnumerable<TSource> source, int count);
可以看到,该扩展方法只接受一个整形的参数,表示跳过的元素数量。
看下面的例子:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace PartitionOperation { class Program { static void Main(string[] args) { int[] source = new int[] { 86, 2, 77, 94, 100, 65, 5, 22, 70, 55, 81, 66, 45 }; // 跳过5个元素 var q = source.Skip(5); foreach (var item in q) { Console.WriteLine(item); } Console.ReadKey(); } } }
结果:
四、SkipWhile操作符
SkipWhile操作符用于从输入序列中跳过满足一定条件指定数量的元素,与TakeWhile操作符类似。来看下SkipWhile操作符的方法定义:
public static IEnumerable<TSource> SkipWhile<TSource>(this IEnumerable<TSource> source, Func<TSource, bool> predicate); public static IEnumerable<TSource> SkipWhile<TSource>(this IEnumerable<TSource> source, Func<TSource, int, bool> predicate);
当SkipWhile操作符被调用时,会将输入序列中的元素走位参数传递给委托predicate,只要predicate的返回值为true,该元素就会被跳过,继续下一个元素,直到遇到一个使predicate返回值为false的元素,此元素以及输入序列中剩余的元素将组合一个新的序列返回。注意后面的不再判断,直接添加到返回序列。
第二个扩展方法的委托里的参数多了个int,还是代表下标,可以根据下标做一些逻辑处理。
看下面的例子:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace PartitionOperation { class Program { static void Main(string[] args) { int[] source = new int[] { 86, 2, 77, 94, 100, 65, 5, 22, 70, 55, 81, 66, 45 }; // 跳过数组中元素值小于100的元素 var q = source.SkipWhile(i => i < 100); foreach (var item in q) { Console.WriteLine(item); } Console.ReadKey(); } } }
结果:
五、使用Take和Skip实现分页
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace PartitionOperation { class Program { static void Main(string[] args) { // 每页显示的条数 int PageSize = 3; // 页数从0开始 int PageNum = 0; int[] source = new int[] { 86, 2, 77, 94, 100, 65, 5, 22, 70, 55, 81, 66, 45 }; while(PageNum*PageSize<source.Length) { // 分页 var query = source.Skip(PageNum * PageSize).Take(PageSize); Console.WriteLine($"输出第{PageNum+1}页记录"); foreach (var item in query) { Console.WriteLine(item); } PageNum++; } Console.ReadKey(); } } }
结果:
栏 目:.NET代码
下一篇:C#利用File方法对文件的操作总结(字节写入和读取)
本文标题:linq中的分区操作符
本文地址:http://www.codeinn.net/misctech/226603.html
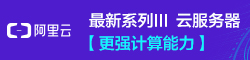
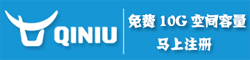