vue filters和directives访问this的问题详解
时间:2023-03-02 09:34:49|栏目:vue|点击: 次
vue filters和directives访问this
记录一次奇葩的需求。
要求自定义一个指令,点击后能跳转指定路由。
directives和filters压根就没法访问this。
脑袋都想破了。
不废话了,上代码。
<template> <div> <div v-join="(divData, that)">tag标签</div> <p>{{divData}}</p> <p>{{divData | sum(that)}}</p> </div> </template> <script> export default { name: 'Main', data(){ return { divData:'传入的值', that: this, filtersData: '过滤器的值' } }, filters: { sum(val, that){ return `${val}和${that.filtersData}` } }, directives: { join: { inserted(el, binding){ }, bind(el, binding){ console.log('------2') el.addEventListener('click', function(){ binding.value.that.$router.push({ path: '/aside' }) }) } } } } </script>
解决方案是在data中定义一个that变量指向this,再将这个变量当做参数传进directives,在directives内部就可以访问到this了,filters同理。
directives所遇小bug
自己在利用vue写todoList的时候遇到一个小bug,记录一下
写个指令,当双击进行编辑todo,让其自动聚焦,结果效果如下,
代码如下:
directives: { focus(el,bindings) { if(bindings.value) { el.focus(); } } }
<input v-focus="todo == t" type="text" v-show="todo == t" v-model="todo.title" @blur="reset" @keyup.13="reset" >
多方查找原因,把自定义v-focus指令放末尾,就好了,代码如下:
<input type="text" v-show="todo == t" v-model="todo.title" @blur="reset" @keyup.13="reset" v-focus="todo == t">
是否自定义指令都应放后面呢?这就需要以后验证了
完整代码如下:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> <link rel="stylesheet" href="../node_modules/bootstrap/dist/css/bootstrap.css"> <style> .del{ text-decoration: line-through; color: #ccc!important; } </style> </head> <body> <div id="app"> <div class="container"> <div class="row"> <div class="col-md-12"> <div class="panel panel-warning"> <div class="panel-heading"> <input type="text" v-model="value" class="form-control" @keydown.enter="add"> </div> <div class="panel-body"> <ul class="list-group"> <li class="list-group-item" @dblclick="change(todo)" v-for="(todo,index) in todos" :key="index"> <div v-show="todo != t" :class="{del: todo.isSelected}"> <input type="checkbox" v-model="todo.isSelected">{{todo.title}} <button class="pull-right btn btn-danger btn-xs" @click="remove(index)">⨂</button> </div> <input type="text" v-show="todo == t" v-model="todo.title" @blur="reset" @keyup.13="reset" v-focus="todo == t"> <!-- 下面是错误代码,可以把上面的注释掉打开下面的对比下 --> <!-- <input v-focus="todo == t" type="text" v-show="todo == t" v-model="todo.title" @blur="reset" @keyup.13="reset" > --> </li> </ul> </div> <div class="panel-footer"> <ul class="nav nav-pills"> <li role="presentation" class="active"><a href="#">全部</a></li> <li role="presentation"><a href="#">已完成</a></li> <li role="presentation"><a href="#">未完成</a></li> </ul> </div> </div> </div> </div> </div> </div> <script src="../node_modules/vue/dist/vue.js"></script> <script> let vm = new Vue({ el:'#app', data:{ todos:[], hash:'complete',//路径切换时 获取的hash值 value:'',// 输入框中需要增加的内容, t:''//当前点击的那一个 }, created(){ //当vue创建时执行的方法 //如果storage有 就用这里的值 没有就是空数组 this.todos = JSON.parse(localStorage.getItem('todos')) || [{isSelected:true,title:'晚上回去睡觉'}]; }, watch:{ //watch默认 只监控一层,例如 todos 可以监控数组的变化,监控不到对象的变化 todos:{ handler(){ localStorage.setItem('todos',JSON.stringify(this.todos)); }, deep:true } }, methods:{ addTodo(){ let todo = {isSelected:false,title:this.value}; this.todos.push(todo); this.value = ''; }, remove(todo){ this.todos = this.todos.filter(item=>todo!==item); }, change(todo){ //todo代表的是我当前点击的是哪一个,存储当前点击的这一项 this.t = todo; }, reset(){ this.t = ''; } }, computed:{ lists(){ if(this.hash === 'complete'){ return this.todos; } if(this.hash === 'finish'){ return this.todos.filter(item=>item.isSelected) } if(this.hash === 'unfinish'){ return this.todos.filter(item=>!item.isSelected) } }, total(){ //求出数组中为false的个数 //将数组中的true全部干掉,求出剩余length return this.todos.filter(item=>!item.isSelected).length; } }, directives:{ //指令,就是操作dom focus(el,bindings){ //bindings中有一个value属性 代表的是指令对应的值v-auto-focus="值" if(bindings.value){ el.focus(); } //console.log(el,bindings); } } }); let listener = () => { let hash = window.location.hash.slice(1) || 'complete'; //如果打开页面没有hash默认是全部 vm.hash = hash; }; listener(); //页面一加载 就需要获取一次hash值,否则可能导致 回到默认hash window.addEventListener('hashchange',listener,false); </script> </body> </html>
上一篇:vue项目ElementUI组件中el-upload组件与图片裁剪功能组件结合使用详解
栏 目:vue
本文标题:vue filters和directives访问this的问题详解
本文地址:http://www.codeinn.net/misctech/226738.html
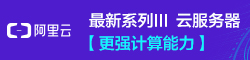
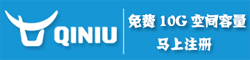