C#实现的简单整数四则运算计算器功能示例
时间:2020-12-15 01:35:48|栏目:.NET代码|点击: 次
本文实例讲述了C#实现的简单整数四则运算计算器功能。分享给大家供大家参考,具体如下:
运行效果图如下:
具体代码如下:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Text; using System.Windows.Forms; namespace 计算器 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } public string num; public int flag;//用于判断输入的操作符 public double num1, num2; private void num0_button_Click(object sender, EventArgs e) { num = num + "0"; num2 = Convert.ToDouble(num); textBox.Text = num; } private void num1_button_Click(object sender, EventArgs e)//重点算法1 { if (textBox.Text == "0") { num = "1"; textBox.Text = Convert.ToString(num); } else { num = num + "1"; num2 = Convert.ToDouble(num); textBox.Text = num; } } private void num2_button_Click(object sender, EventArgs e) { if (textBox.Text == "0") { num = "2"; textBox.Text = Convert.ToString(num); } else { num = num + "2"; num2 = Convert.ToDouble(num); textBox.Text = num; } } private void num3_button_Click(object sender, EventArgs e) { if (textBox.Text == "0") { num = "3"; textBox.Text = Convert.ToString(num); } else { num = num + "3"; num2 = Convert.ToDouble(num); textBox.Text = num; } } private void num4_button_Click(object sender, EventArgs e) { if (textBox.Text == "0") { num = "4"; textBox.Text = Convert.ToString(num); } else { num = num + "4"; num2 = Convert.ToDouble(num); textBox.Text = num; } } private void num5_button_Click(object sender, EventArgs e) { if (textBox.Text == "0") { num = "5"; textBox.Text = Convert.ToString(num); } else { num = num + "5"; num2 = Convert.ToDouble(num); textBox.Text = num; } } private void num6_button_Click(object sender, EventArgs e) { if (textBox.Text == "0") { num = "6"; textBox.Text = Convert.ToString(num); } else { num = num + "6"; num2 = Convert.ToDouble(num); textBox.Text = num; } } private void num7_button_Click(object sender, EventArgs e) { if (textBox.Text == "0") { num = "7"; textBox.Text = Convert.ToString(num); } else { num = num + "7"; num2 = Convert.ToDouble(num); textBox.Text = num; } } private void num8_button_Click(object sender, EventArgs e) { if (textBox.Text == "0") { num = "8"; textBox.Text = Convert.ToString(num); } else { num = num + "8"; num2 = Convert.ToDouble(num); textBox.Text = num; } } private void num9_button_Click(object sender, EventArgs e) { if (textBox.Text == "0") { num = "9"; textBox.Text = Convert.ToString(num); } else { num = num + "9"; num2 = Convert.ToDouble(num); textBox.Text = num; } } private void add_button_Click(object sender, EventArgs e)//重点算法2 { if (textBox.Text.Length > 0) { num1 = Convert.ToDouble(textBox .Text); num = ""; flag = 1; textBox.Text = ""; textBox.Focus(); } } private void dev_button_Click(object sender, EventArgs e) { if (textBox.Text.Length > 0) { num1 = Convert.ToDouble(textBox.Text); num = ""; flag = 2; textBox.Text = ""; textBox.Focus(); } } private void mul_button_Click(object sender, EventArgs e) { if (textBox.Text.Length > 0) { num1 = Convert.ToDouble(textBox.Text); num = ""; flag = 3; textBox.Text = ""; textBox.Focus(); } } private void chu_button_Click(object sender, EventArgs e) { if (textBox.Text.Length > 0) { num1 = Convert.ToDouble(textBox.Text); num = ""; flag = 4; // textBox.Text = ""; textBox.Focus(); } } private void equ_button_Click(object sender, EventArgs e) { switch (flag) { case 1: textBox.Text = Convert.ToString(num1+Convert .ToDouble(num));//重点算法3 num2 = Convert.ToDouble(textBox .Text); break; case 2: textBox.Text = Convert.ToString(num1 - Convert.ToDouble(num)); num2 = Convert.ToDouble(textBox.Text); break; case 3: textBox.Text = Convert.ToString(num1 * Convert.ToDouble(num)); num2 = Convert.ToDouble(textBox.Text); break; case 4: textBox.Text = Convert.ToString(num1 / Convert.ToDouble(num)); num2 = Convert.ToDouble(textBox.Text); break; } } private void re_button_Click(object sender, EventArgs e) { num = ""; textBox.Text = "0"; } } }
PS:这里再为大家推荐几款计算工具供大家进一步参考借鉴:
在线一元函数(方程)求解计算工具:
http://tools.jb51.net/jisuanqi/equ_jisuanqi
科学计算器在线使用_高级计算器在线计算:
http://tools.jb51.net/jisuanqi/jsqkexue
在线计算器_标准计算器:
http://tools.jb51.net/jisuanqi/jsq
更多关于C#相关内容感兴趣的读者可查看本站专题:《C#数据结构与算法教程》、《C#程序设计之线程使用技巧总结》、《C#常见控件用法教程》、《WinForm控件用法总结》、《C#数组操作技巧总结》及《C#面向对象程序设计入门教程》
希望本文所述对大家C#程序设计有所帮助。
栏 目:.NET代码
下一篇:asp将本地的文件上传到服务器
本文标题:C#实现的简单整数四则运算计算器功能示例
本文地址:http://www.codeinn.net/misctech/33036.html
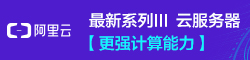
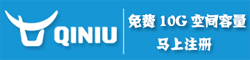