Scala IF...ELSE语句
下面是一个典型的决策中IF...ELSE结构的一般形式使用在大多数的编程语言中:
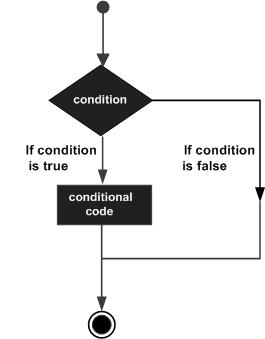
if 语句:
if 语句包含一个布尔表达式后跟一个或多个语句。
语法:
一个 if 语句的语法:
if(Boolean_expression) { // Statements will execute if the Boolean expression is true }
如果布尔表达式的值为true,那么if语句里面的代码模块将被执行。如果不是这样,第一组码if语句结束后(右大括号后)将被执行。
示例:
object Test { def main(args: Array[String]) { var x = 10; if( x < 20 ){ println("This is if statement"); } } }
这将产生以下输出结果:
C:/>scalac Test.scala C:/>scala Test This is if statement C:/>
if...else语句:
if语句可以跟着一个可选的else语句,当 else 块执行时,布尔表达式条件是假的。
语法:
if...else的语法是:
if(Boolean_expression){ //Executes when the Boolean expression is true }else{ //Executes when the Boolean expression is false }
示例:
object Test { def main(args: Array[String]) { var x = 30; if( x < 20 ){ println("This is if statement"); }else{ println("This is else statement"); } } }
这将产生以下结果:
C:/>scalac Test.scala C:/>scala Test This is else statement C:/>
if...else if...else语句:
if语句可以跟着一个可选的else if ... else语句,这是非常有用的使用 if...else if如果测试各种条件声明。
当使用 if , else if , else 语句有几点要牢记。
-
if可以有零或一个else,它必须跟在else if后面。
-
一个if 可以有零到多个else if,并且它们必须在else之前。
-
一旦一个 else if 匹配成功,剩余的else if或else不会被测试匹配。
语法:
if...else if...else的语法是:
if(Boolean_expression 1){ //Executes when the Boolean expression 1 is true }else if(Boolean_expression 2){ //Executes when the Boolean expression 2 is true }else if(Boolean_expression 3){ //Executes when the Boolean expression 3 is true }else { //Executes when the none of the above condition is true. }
示例:
object Test { def main(args: Array[String]) { var x = 30; if( x == 10 ){ println("Value of X is 10"); }else if( x == 20 ){ println("Value of X is 20"); }else if( x == 30 ){ println("Value of X is 30"); }else{ println("This is else statement"); } } }
这将产生以下结果:
C:/>scalac Test.scala C:/>scala Test Value of X is 30 C:/>
if ... else语句嵌套:
它始终是合法的嵌套 if-else 语句,这意味着可以使用一个 if 或 else if 在另一个if 或 else if 语句中。
语法:
语法嵌套 if...else 如下:
if(Boolean_expression 1){ //Executes when the Boolean expression 1 is true if(Boolean_expression 2){ //Executes when the Boolean expression 2 is true } }
可以嵌套else if...else在if语句中,反之也可以。
示例:
object Test { def main(args: Array[String]) { var x = 30; var y = 10; if( x == 30 ){ if( y == 10 ){ println("X = 30 and Y = 10"); } } } }
这将产生以下结果:
C:/>scalac Test.scala C:/>scala Test X = 30 and Y = 10 C:/>
本站文章除注明转载外,均为本站原创或编译
欢迎任何形式的转载,但请务必注明出处,尊重他人劳动共创优秀实例教程
转载请注明:文章转载自:代码驿站 [http:/www.codeinn.net]
本文标题:Scala IF...ELSE语句
本文地址:http://www.codeinn.net/scala/1204.html
欢迎任何形式的转载,但请务必注明出处,尊重他人劳动共创优秀实例教程
转载请注明:文章转载自:代码驿站 [http:/www.codeinn.net]
本文标题:Scala IF...ELSE语句
本文地址:http://www.codeinn.net/scala/1204.html